10 JavaScript Array Methods That Will Make You a Better Programmer
November 24, 2024
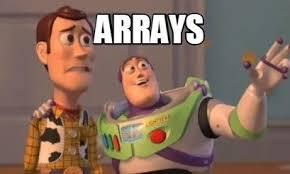
As a JavaScript developer, mastering array methods is crucial for writing clean and efficient code. In this guide, we’ll explore 10 powerful array methods that will significantly improve your programming skills.
1. map() - Transform Array Elements
The map()
method creates a new array by transforming each element using a callback function.
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(num => num 2);
// Result: [2, 4, 6, 8]
// Real-world example: Formatting API data
const users = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' }
];
const userNames = users.map(user => user.name);
// Result: ['John', 'Jane']
2. filter() - Select Specific Elements
Use filter()
to create a new array containing elements that pass a test condition.
const numbers = [1, 2, 3, 4, 5, 6];
const evenNumbers = numbers.filter(num => num % 2 === 0);
// Result: [2, 4, 6]
// Real-world example: Filtering active users
const users = [
{ id: 1, name: 'John', active: true },
{ id: 2, name: 'Jane', active: false }
];
const activeUsers = users.filter(user => user.active);
// Result: [{ id: 1, name: 'John', active: true }]
3. reduce() - Accumulate Values
The reduce()
method combines array elements into a single value.
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
// Result: 10
// Real-world example: Calculating total price
const cart = [
{ item: 'Book', price: 20 },
{ item: 'Pen', price: 5 }
];
const total = cart.reduce((sum, item) => sum + item.price, 0);
// Result: 25
4. find() - Locate Specific Elements
find()
returns the first element that matches a condition.
const users = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' }
];
const jane = users.find(user => user.name === 'Jane');
// Result: { id: 2, name: 'Jane' }
5. some() - Check for Any Matches
Use some()
to test if at least one element meets a condition.
const numbers = [1, 2, 3, 4, 5];
const hasEven = numbers.some(num => num % 2 === 0);
// Result: true
// Real-world example: Checking permissions
const userRoles = ['user', 'editor', 'viewer'];
const isAdmin = userRoles.some(role => role === 'admin');
// Result: false
6. every() - Verify All Elements
every()
checks if all elements satisfy a condition.
const numbers = [2, 4, 6, 8];
const allEven = numbers.every(num => num % 2 === 0);
// Result: true
// Real-world example: Form validation
const formFields = [
{ name: 'email', valid: true },
{ name: 'password', valid: true }
];
const isFormValid = formFields.every(field => field.valid);
// Result: true
7. includes() - Check Element Existence
The includes()
method checks if an array contains a specific value.
js
const fruits = ['apple', 'banana', 'orange'];
const hasBanana = fruits.includes('banana');
// Result: true
// Real-world example: Permission checking
const allowedRoles = ['admin', 'editor'];
const userRole = 'editor';
const hasAccess = allowedRoles.includes(userRole);
// Result: true
8. slice() - Extract Array Portions
Use slice()
to create a new array containing selected elements.
const numbers = [1, 2, 3, 4, 5];
const subset = numbers.slice(1, 4);
// Result: [2, 3, 4]
// Real-world example: Pagination
const posts = ['Post1', 'Post2', 'Post3', 'Post4', 'Post5'];
const pageSize = 2;
const page1 = posts.slice(0, pageSize);
// Result: ['Post1', 'Post2']
9. flatMap() - Map and Flatten Results
flatMap()
combines map()
and flat()
operations for nested arrays.
const sentences = ['Hello world', 'How are you'];
const words = sentences.flatMap(sentence => sentence.split(' '));
// Result: ['Hello', 'world', 'How', 'are', 'you']
// Real-world example: Processing nested data
const orders = [
{ items: ['book', 'pen'] },
{ items: ['notebook'] }
];
const allItems = orders.flatMap(order => order.items);
// Result: ['book', 'pen', 'notebook']
10. at() - Access Elements with Relative Indexing
The at()
method provides a cleaner way to access array elements, especially for negative indices.
js
const numbers = [1, 2, 3, 4, 5];
const lastItem = numbers.at(-1);
// Result: 5
// Real-world example: Accessing recent items
const recentPosts = ['Post1', 'Post2', 'Post3'];
const mostRecent = recentPosts.at(-1);
// Result: 'Post3'
Best Practices and Tips
- Chain Methods: Combine multiple array methods for complex operations:
const numbers = [1, 2, 3, 4, 5, 6];
const sumOfDoubledEvens = numbers
.filter(num => num % 2 === 0)
.map(num => num 2)
.reduce((sum, num) => sum + num, 0);
// Result: 24 (22 + 42 + 62)
- Avoid Mutation: Most of these methods return new arrays, preserving immutability:
// Good practice
const original = [1, 2, 3];
const doubled = original.map(num => num 2);
// Avoid direct mutation
// original.forEach((num, i) => original[i] = num 2);
- Consider Performance: For large arrays, consider using traditional loops if performance is critical.
Conclusion
These array methods are essential tools in modern JavaScript development. They help write more declarative, readable, and maintainable code. Practice using these methods in your projects, and you’ll notice significant improvements in your code quality and productivity.
Remember, the key to mastering these methods is understanding when to use each one and how they can be combined to solve complex problems efficiently.